티스토리 뷰
1. 아래와 같이 프로그래밍 하시오.
interface Shape
- double getArea();
- void setWidth(double width)
- void setHeight(double height)
삼각형 , 사각형 객체를 위의 인터페이스를 구현하여,
appCTX4에 객체 생성후 Pencile 과 같이 다형성이 적용되도록 Main을 짜시오.
아래와 같이 패키지 생성(shape가 이미 있어서 shape2로 생성)
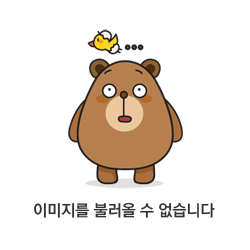
interface Shape 생성
1
2
3
4
5
6
7
8
|
package com.javalec.ex.shape2;
public interface Shape {
// 앞에 abstract가 생략된 상태
double getArea();
void setWidth(double width);
void setHeight(double height);
}
|
cs |
Rectangle.class
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
|
package com.javalec.ex.shape2;
public class Rectangle implements Shape {
private double width;
private double height;
public Rectangle() {
}
@Override
public void setWidth(double width) {
this.width = width;
}
@Override
public void setHeight(double height) {
this.height = height;
}
public double getWidth() {
return width;
}
public double getHeight() {
return height;
}
@Override
public double getArea() {
return width * height;
}
}
|
cs |
Triangle.class
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
|
package com.javalec.ex.shape2;
public class Triangle implements Shape {
private double width;
private double height;
public Triangle() {
}
@Override
public void setWidth(double width) {
this.width = width;
}
@Override
public void setHeight(double height) {
this.height = height;
}
public double getWidth() {
return width;
}
public double getHeight() {
return height;
}
@Override
public double getArea() {
return (width * height) / 2;
}
}
|
cs |
appCTX4.xml
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
|
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<!-- 마지막에 넣는 클래스명만(Triangle)
수정하면 ShapeAreaMain.java에서 출력 결과가 바뀐다!!
main에서 직접 객체를 다시 생성해 주지 않아도 된다.
소스코드를 수정하지 않아도 된다!! 결과물은 차이가 없어보이지만
이게 훨씬 더 효율적이고 오류가 적은 방법이다.
그래서 spring이 유용한 것이다! -->
<bean id="shape" class="com.javalec.ex.shape2.Triangle">
<property name="width">
<value>10</value>
</property>
<property name="height">
<value>20</value>
</property>
</bean>
</beans>
<!-- 개발자, 경제적 관점에서는 사실 소스코드가 복잡해도 일자리 많이 생기는게 더 좋....
하지만 사업자 입장에서는 유지보수비용이 많이 들어서 별로....
스프링을 사용하면 직접 소스코드를 수정하지 않아도 출력 결과를 바꿀수 있게 되니까
개발자가 아니라도 쉽게 수정할 수 있어 유지, 보수에 필요한 인력과 비용이 줄어든다.
ㅋㅋㅋ그래서 유지보수하는 엔지니어들이 쉽고, 개발자만큼의 실력이 없어도 된다?!!?-->
<!-- 결국 최대한 소스코드를 직접 수정하지 않도록 짜는 사람이 실력 좋은 개발자... -->
|
cs |
ShapeAreaMain.class
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
|
package com.javalec.ex.shape2;
import org.springframework.context.support.AbstractApplicationContext;
import org.springframework.context.support.GenericXmlApplicationContext;
public class ShapeAreaMain {
public static void main(String[] args) {
String config = "classpath:appCTX4.xml";
AbstractApplicationContext ctx = new GenericXmlApplicationContext(config);
// 최고 부모를 넣어준다...
Shape shape = ctx.getBean("shape", Shape.class);
System.out.println("넓이는 " + shape.getArea() + "입니다.");
// 이런 방식으로도 가능하다....
shape.setHeight(100);
shape.setWidth(100);
System.out.println("넓이는 " + shape.getArea() + "입니다.");
ctx.close();// 꼭 필요한 건 아니지만 해주면 좋다..?
// 이걸 사용하면 출력 결과물 밑에 빨간 문구들이 추가로 나오는데
// 오류가 아니라 close 문구이다.
}
}
|
cs |
2. javascript 에서, 데이터 타입 종류와 변수 선언 방법은?
참조형도 있지만 우선 기본형 6가지만....
우선 javascript에서 변수를 선언할 때는 데이터 타입에 상관없이 모두 var를 사용한다.
- 기본 타입(Primitive Type)
1) 숫자(number) : 정수, 실수 등 숫자는 모두 Number 타입에 속한다. 다만 자바와는 다르게 0으로 나누거나 계산이 불가능할 경우 출력되는 문구가 있다.
0으로 양수를 나누면 Infinity 출력, 0으로 음수를 나누면 -Infinity 출력, 계산할 수 없을 경우에는 NaN를 출력한다.
1
2
3
4
5
6
|
var num2 = 10/0;
console.log(num2) // +Infinity
var num3 = -10/0;
console.log(num3) // -Infinity
var num4 = 1 * 'a';
console.log(num4) // NaN
|
cs |
2) 문자열(string) : 문자, 문자열은 String 타입으로 문자열을 나타낼 때는 ""와 ''둘 다 사용 가능하다.
1
2
3
4
5
6
|
var str = "가나다라마바사";
console.log(str);
console.log(str + 'ABCDEFG'); //가다나다라마바사ABCDEFG
// 문자열 + 문자열은 두 문자열을 붙여서 출력
console.log(str + '\n\tABCDEFG\"'); // ABCDEFG"
// \사용하면 java 이스케이프문자와 같음..
|
cs |
3) 불린값(boolean) : true, false(참, 거짓)을 나타내는 데이터 타입.
4) undefined : 값이 할당되지 않았을 때, 자바스크립트 엔진이 암묵적으로 초기화하는 값
1
2
|
var none;
console.log(none); // undefined
|
cs |
5) object(객체) : 복잡한 형태의 값들이 묶여있는 형태(자바의 class를 생각해보자)....null값도 object 타입이다. object를 표현 할 때는 { }를 사용한다.
var dog = { name: "해피", age: 3 };
6) 심벌(symbol) : ES6부터 새로 생긴 데이터 타입. 변경불가능한 유일한 값을 생성할 때 사용한다.
1
2
|
var sym = Symbol("javascript");
console.log(sym); // Symbol(javascript)
|
cs |
3. javascript 에서 아래의 함수는?
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
|
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script>
alert("Hello Javascript!!"); //; 안써도 상관없지만 이왕이면 무조건 쓰자!
// 'Hello Javascript'로 써도 상관없다.
// 다만 출력을 ''까지 하고 싶다면 ""안에 ''를 써서
// "'Hello Javascript'"로 써야한다.
// (팝업창 내용, 입력 칸에 들어갈 내용.. placeholder같은 역할-아무것도 입력안하면 이게 디폴트 값)
var inpuPro = prompt("출력창입니다.", "문장을 입력하세요.");
alert(inpuPro);
var inpuCon = confirm("진행하겠습니다.");
// 확인, 취소 버튼이 나타난다. 확인을 누르면 true, 취소를 누르면 false 출력
alert(inpuCon);
// 자바랑 다르게 자료형을 정해주고 변수 선언을 하는 것이 아니라
// 어떤 데이터타입이라도 var하나로 변수 선언(다만 일단 데이터타입 종류 자체는 6가지 있다)
console.log(inpuPro); // 입력한 값 출력
console.log(inpuCon); // 확인 true, 취소 false 출력
</script>
</head>
<body>
</body>
</html>
|
cs |
-alret : 팝업창을 띄운다.
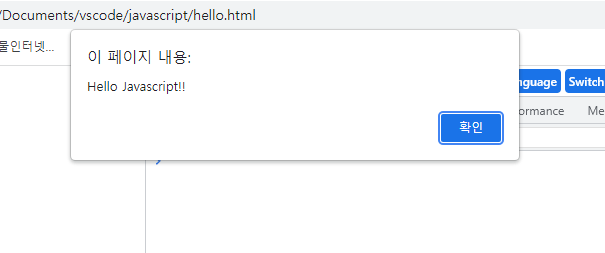
-prompt : 입력할 수 있는 출력창.
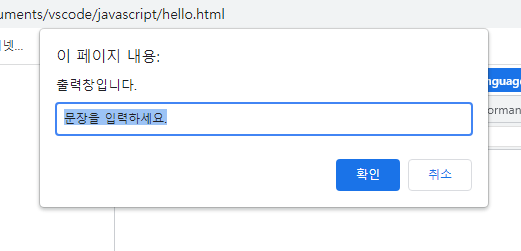
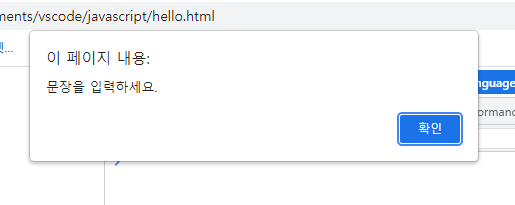
입력한 값을 다시 출력
-confirm : 지정한 문구와 함께 확인, 취소 버튼이 나타난다. 이 때 확인을 누르면 true, 취소를 누르면 false를 반환한다.
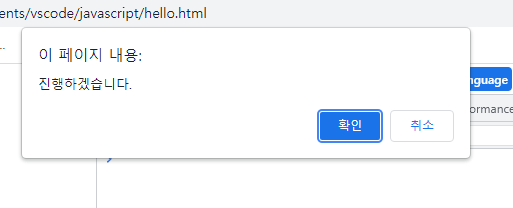
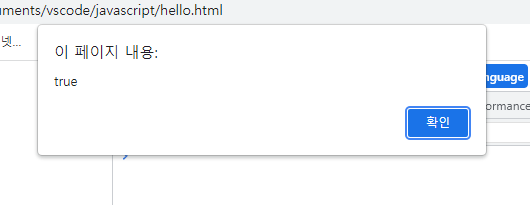
F12 눌렀을 때 console창
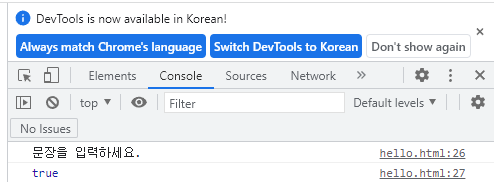
54번
ThreadCount threadCount = new ThreadCount();
threadCount.start();
String input = JOptionPane.showInputDialog("아무 값이나 입력하세요.");
System.out.println("입력하신 값은 " + input + "입니다.");
10 9 8 7 6 ... 이 1초마다 실행 되도록 쓰레를 완성하시오.
import javax.swing.JOptionPane;
class ThreadCount extends Thread{
@Override
public void run() {
for(int i = 10; i > 0; i--) {
System.out.println(i);
try {
Thread.sleep(1000);
} catch (Exception e) {
}
}
}
}
public class TimeTest {
public static void main(String[] args) {
ThreadCount threadCount = new ThreadCount();
threadCount.start();
String input = JOptionPane.showInputDialog("아무 값이나 입력하세요.");
System.out.println("입력하신 값은 " + input + "입니다.");
}
}
55번
html - 메뉴 4개 구성
html 12강 css속성 에 있습니다.
12-2. font-style, font-weight, line-height 속성
<!DOCTYPE html>
<html lang="en" xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta charset="utf-8" />
<title></title>
<style>
#nav {
width:800px;
margin:0 auto;
border:1px solid #cccccc;
overflow:hidden;
}
#nav div {
width:150px;
height:100px;
line-height:100px;
float:left;
text-align:center;
font-size:1.5em;
border-top:1px solid #cccccc;
border-bottom:1px solid #cccccc;
margin:5px;
}
a {
text-decoration:none;
color:#282828;
}
</style>
</head>
<body>
<div id="nav">
<div><a href="#">menu1</a></div>
<div><a href="#">menu2</a></div>
<div><a href="#">menu3</a></div>
<div><a href="#">menu4</a></div>
<div><a href="#">menu5</a></div>
</div>
</body>
</html>
'수업문제' 카테고리의 다른 글
[문제] 12월 15일 (pom.xml, @RequestMapping, Model, maven repository, mutable 함수 , immutable 함수) (0) | 2021.12.15 |
---|---|
[문제] 12월 14일 (자바 스크립트, Number 함수, String 함수, 데이터 타입) (0) | 2021.12.14 |
[문제] 12월 10일 (DI, IOC, IOC 컨테이너, Spring 입문) (0) | 2021.12.10 |
[문제] 12월 09일 (게시판 템플릿 적용하기) (0) | 2021.12.09 |
- Total
- Today
- Yesterday
- JSP
- el
- 채팅
- 쿠키
- Request
- 예외처리
- Session
- toString
- 참조형
- 세션
- 입출력
- 부트스트랩
- equals
- hashset
- abstract
- 진척도 70번
- 프로토콜
- response
- Servlet
- object
- 제네릭
- 쓰레드
- 사칙연산 계산기
- TreeSet
- exception
- Generic
- string
- 래퍼 클래스
- SOCKET
- compareTo
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | ||||
4 | 5 | 6 | 7 | 8 | 9 | 10 |
11 | 12 | 13 | 14 | 15 | 16 | 17 |
18 | 19 | 20 | 21 | 22 | 23 | 24 |
25 | 26 | 27 | 28 | 29 | 30 | 31 |